목록Solved (19)
Dev.J
이름과 전화번호를 입력받아 검색하는 프로그램을 HashMap 을 이용하여 구현하시오. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 import java.util.HashMap; import java.util.Scanner; import java.util.Set; import java.util.Iterator; public class Assignment12_3 { public static void main(String[] args) { HashMap pbook = new HashMap(); System.out.print("입력하려는 데이터의 수는..
3개의 나라이름과 인구를 입력받아 HashMap에 저장하고. 가장 인구가 많은 나라를 검색하여 출력하는 프로그램을 완성하시오. map의 키값들을 가져와서(keySet() 을 이용) Iterator를 사용하여 제일 큰 값을 찾는다. 1) keySet()메소드로 Set컬렉션을 얻은 후, 반복자로 하나씩 get() 통해 값을 가져온다. Set keys = map.keySet(); Iterator it = keys.iterator(); while (it.hasNext()) { String key = it.next(); System.out.println(String.format("키 : %s, 값 : %s", key, map.get(key))); } for (String key : map.keySet())..
다음 3개의 데이터를 입력받아 프로그램을 완성하시오. (java, 자바) (school, 학교) (map, 지도) quit를 입력하면 종료한다. - Hashmap 사용 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 import java.util.HashMap; import java.util.Scanner; public class Assignment12_1 { public static void main(String[] args) { HashMap word = new HashMap(); word.put("java","자바"); //값 추..
배열을 이용하여 극장 예약시스템을 작성하세요. 좌석수가 5개이고 사용자가 예약을 하려면 먼저 좌석배치표를 보여준다. 예약이 끝난 좌석은 1로, 예약이 안된 좌석은 0으로 나타낸다. 예약되었는지를 출력해주고, 만석이면 예약을 종료하도록 한다. public class Assignment6_2 { // 첫 풀이때 문제점은 예약하는 if 문과 만석 판단하는 if 문이 분리되지 않았음 public static void main(String[] args) { Scanner sc = new Scanner(System.in); int num1 = 0; // 예약좌석번호 int[] seat = new int[5]; seat[0] = 0; seat[1] = 0; seat[2] = 0; seat[3] = 0;..
Customer 클래스 class Customer { protected String customerID; //고객아이디 protected String customerName; //고객이름 protected String customerGrade; //고객등급 public String getCustomerID() { return customerID; } public void setCustomerID(String customerID) { this.customerID = customerID; } public String getCustomerName() { return customerName; } public void setCustomerName(String customerName) { this.customerN..
다음과 같은 설계로 인터페이스를 구현하세요. Device 추상클래스 public abstract class Device { public abstract void print(); } Printer 클래스 extends Device implements Connectable public class Printer extends Device implements Connectable { public void print(){ System.out.println("프린터입니다."); } public void connect() { System.out.println(name +"와 프린터를 연결합니다."); } } UsbMem 클래스 extends Device implements Connectable public cla..
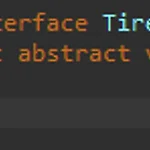
자동차는 타이어 인터페이스를 이용해서 한국타이어와 금호 타이어를 사용한다. Car객체의 run()에서 타이어 인터페이스에 선언된 roll()을 호출한다. overriding된 각 roll() 메소드에서 회사의 이름 타이어를 출력한다. Tire 인터페이스 public interface Tire { public abstract void roll(); } KumhoTire 클래스 implements Tire public class KumhoTire implements Tire { public void roll() { System.out.println("금호 타이어가 굴러갑니다."); } } HankookTire 클래스 implements Tire public class HankookTire impl..
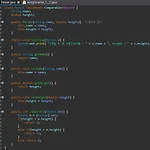
Person은 Comparable 인터페이스를 구현하고 이 인터페이스를 이용하여 가장 키 큰 사람 의 이름을 반환하는 메소드 getMaximum(Person[] array)를 구현하는 프로그램을 완성하세요. Person 클래스 class Person implements Comparable { String name; double height; public Person(String name, double height){ //생성자 정의 this.name = name; this.height = height; } static void toString(Person o) { System.out.print("[제일 키 큰 사람]\n이름 : " + o.name + ", height : " + o.height); }..
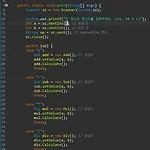
사칙연산을 하는 계산기를 완성하세요. calculate() : 추상메소드 setValue() : 피연산자 설정 Calc 클래스 public abstract class Calc { int a; int b; void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말! this.a = a; this.b = b; } abstract void Calculate(); } Add 클래스 public class Add extends Calc { void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말! this.a = a; this.b = b; } void Calculate() { System.out.p..
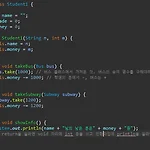
학생이 버스나 지하철을 타고 학교에 가는 것을 객체 지향 프로그램으로 구현한다. 제시하지 않은 것은 임의로 추가하여 구현. 학생클래스(Student) 멤버변수 : 이름(name), 학년(grade), 가진 돈(money) 메소드 버스탄다(takeBus) : 버스를 타면 1000원을 지불 지하철탄다(takeSubway) : 지하철을 타면 1500원을 지불 학생의 현재 정보(showInfo) : 이름과 남은 돈을 출력 버스클래스(Bus) 멤버변수 : 버스번호(busNumber), 승객수(passengerCount), 버스 수입(money) 버스 번호를 매개변수로 받는 생성자 메소드 take : 승객 한명이 버스를 탄 경우. 요금을 매개변수로 받고 승객수와 버스 수입 증가 ..