Dev.J
[JAVA] 문제 4-1 Interface 사칙연산 계산기 본문
사칙연산을 하는 계산기를 완성하세요.
calculate() : 추상메소드
setValue() : 피연산자 설정
Calc 클래스
public abstract class Calc {
int a;
int b;
void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말!
this.a = a;
this.b = b;
}
abstract void Calculate();
}
Add 클래스
public class Add extends Calc {
void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말!
this.a = a;
this.b = b;
}
void Calculate() {
System.out.println(a + " " + b + " - " +(a + b));
}
}
Sub 클래스
public class Sub extends Calc {
void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말!
this.a = a;
this.b = b;
}
void Calculate() {
System.out.println(a + " " + b + " - " +(a - b));
}
}
Mul 클래스
public class Mul extends Calc {
void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말!
this.a = a;
this.b = b;
}
void Calculate() {
System.out.println(a + " " + b + " - " +(a * b));
}
}
Div 클래스
public class Div extends Calc {
void setValue(int a, int b) { // 피연산자 설정 > this.a = a 를 하라는 말!
this.a = a;
this.b = b;
}
void Calculate() {
System.out.println(a + " " + b + " - " +(a/b));
}
}
CalcTest 클래스
import java.util.*;
public class CalcTest {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.printf("두 정수와 연산자를 입력하세오. (ex. 34 5 +)");
int a = sc.nextInt(); // 숫자 1
int b = sc.nextInt(); // 숫자 2
String op = sc.next(); // operation 연산
sc.close();
switch (op) {
case "+":
Add add = new Add(); // 생성자
add.setValue(a, b);
add.Calculate();
break;
case "-":
Sub sub = new Sub(); // 생성자
sub.setValue(a, b);
sub.Calculate();
break;
case "*":
Mul mul = new Mul(); // 생성자
mul.setValue(a, b);
mul.Calculate();
break;
case "/":
Div div = new Div(); // 생성자
div.setValue(a, b);
div.Calculate();
break;
}
}
}
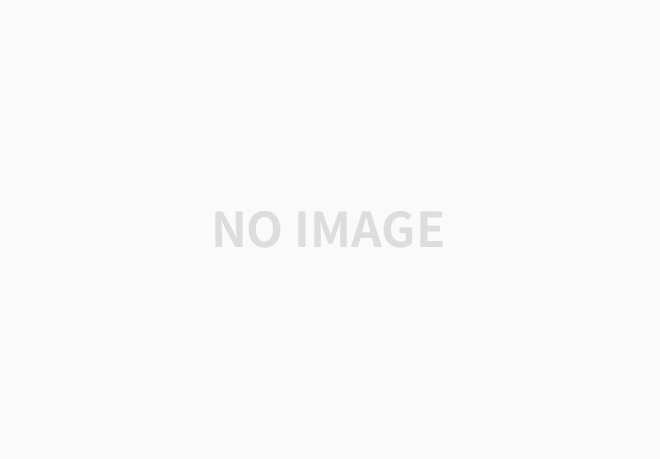
'Solved' 카테고리의 다른 글
[JAVA] 문제 4-5 Interface / 사용된 회사의 이름의 타이어를 출력 (0) | 2021.09.06 |
---|---|
[JAVA] 문제 4-3 Interface / 인터페이스를 이용하여 가장 키 큰 사람 의 이름을 반환 (0) | 2021.09.06 |
[JAVA] 문제 4-4 학생이 버스나 지하철을 타고 학교에 가는 것을 객체 지향 프로그램으로 구현 (0) | 2021.09.05 |
[JAVA] 문제 4-2 배열 상속/Food,Melon (0) | 2021.09.05 |
[JAVA] 문제 3-2, 3-3 객체배열과 상속 (0) | 2021.09.05 |